ตัวอย่างที่ 1 ใช้ NuSoap
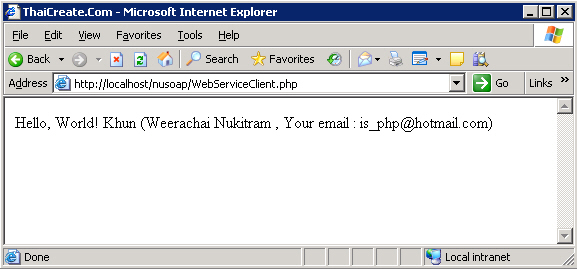
Download Library ของ NuSoap
หรือจะดาวน์โหลดได้จากในส่วนล่างของบทความนี้
สำหรับการสร้าง Web Service ในตัวอย่างนี้จะแบ่งออกเป็น 2 ประเภทคือ
- Web Service ฝั่ง Server ที่ให้บริการ
- Web Service ฝั่ง Client ที่เรียกใช้ Web Service ฝั่ง Server
Web Service ฝั่ง Server
WebServiceServer.php
01.
<?
02.
require_once
(
"lib/nusoap.php"
);
03.
04.
//Create a new soap server
05.
$server
=
new
soap_server();
06.
07.
//Define our namespace
08.
$namespace
=
"http://localhost/nusoap/index.php"
;
09.
$server
->wsdl->schemaTargetNamespace =
$namespace
;
10.
11.
//Configure our WSDL
12.
$server
->configureWSDL(
"HelloWorld"
);
13.
14.
// Register our method and argument parameters
15.
$varname
=
array
(
16.
'strName'
=>
"xsd:string"
,
17.
'strEmail'
=>
"xsd:string"
18.
);
19.
$server
->register(
'HelloWorld'
,
$varname
,
array
(
'return'
=>
'xsd:string'
));
20.
21.
function
HelloWorld(
$strName
,
$strEmail
)
22.
{
23.
return
"Hello, World! Khun ($strName , Your email : $strEmail)"
;
24.
}
25.
26.
// Get our posted data if the service is being consumed
27.
// otherwise leave this data blank.
28.
$POST_DATA
= isset(
$GLOBALS
[
'HTTP_RAW_POST_DATA'
]) ?
$GLOBALS
[
'HTTP_RAW_POST_DATA'
] :
''
;
29.
30.
// pass our posted data (or nothing) to the soap service
31.
$server
->service(
$POST_DATA
);
32.
exit
();
33.
?>
จาก Code มีการสร้าง Service ชื่อว่า HelloWorld และประกอบด้วย Method ชื่อว่า HelloWorld รับค่า argument 2 ตัวคือ $strName และ $strName และทำการ return return "Hello, World! Khun ($strName , Your email : $strEmail)";
ในไฟล์นี้จะจัดเก็บไว้ในโฟเดอร์ \nusoap\WebServiceServer.php
ทดสอบการรัน Web Service
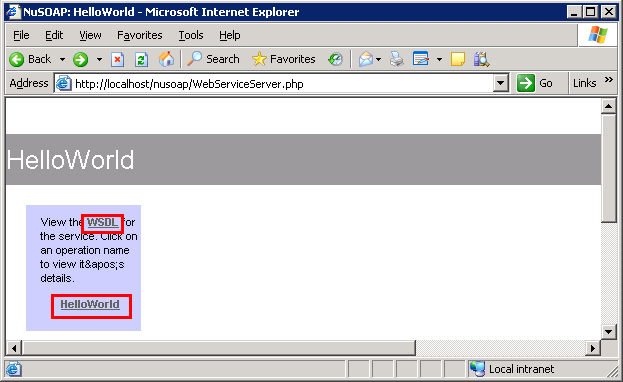
ในตัวอย่างจะเห็น URL ของ Web Service ประกอบด้วย Method ชื่อว่า HellowWorld ให้คลิกที่ WSDL เพื่อดูรูปแบบของ XML
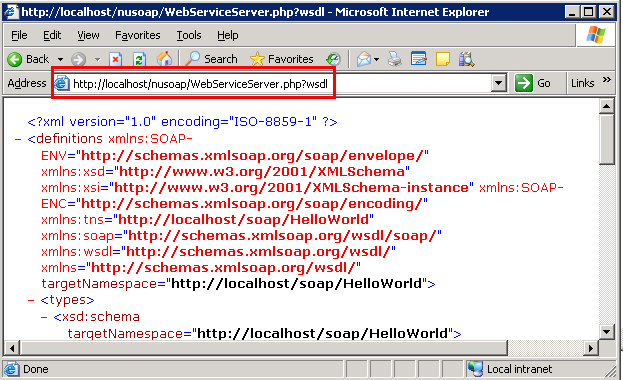
เมื่อรัน URL ของ Web Service ตามด้วย ?wsdl จะได้ XML ที่ประกอบด้วย Format ของ wsdl ดังรูป ซึ่งตอนที่นำไปใช้งานจริง ๆ เราจะใช้ URL นี้
รูปแบบ XML ที่ได้
01.
<?xml version=
"1.0"
encoding=
"ISO-8859-1"
?>
02.
<definitions xmlns:SOAP-ENV=
"http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd=
"http://www.w3.org/2001/XMLSchema"
xmlns:xsi=
"http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC=
"http://schemas.xmlsoap.org/soap/encoding/"
xmlns:tns=
"http://localhost/soap/HelloWorld"
xmlns:soap=
"http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:wsdl=
"http://schemas.xmlsoap.org/wsdl/"
xmlns=
"http://schemas.xmlsoap.org/wsdl/"
targetNamespace=
"http://localhost/soap/HelloWorld"
>
03.
<types>
04.
<xsd:schema targetNamespace=
"http://localhost/soap/HelloWorld"
>
05.
<xsd:import namespace=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
06.
<xsd:import namespace=
"http://schemas.xmlsoap.org/wsdl/"
/>
07.
</xsd:schema>
08.
</types>
09.
<message name=
"HelloWorldRequest"
>
10.
<part name=
"strName"
type=
"xsd:string"
/>
11.
<part name=
"strEmail"
type=
"xsd:string"
/>
12.
</message>
13.
<message name=
"HelloWorldResponse"
>
14.
<part name=
"return"
type=
"xsd:string"
/>
15.
</message>
16.
<portType name=
"HelloWorldPortType"
>
17.
<operation name=
"HelloWorld"
>
18.
<input message=
"tns:HelloWorldRequest"
/>
19.
<output message=
"tns:HelloWorldResponse"
/>
20.
</operation>
21.
</portType>
22.
<binding name=
"HelloWorldBinding"
type=
"tns:HelloWorldPortType"
>
23.
<soap:binding style=
"rpc"
transport=
"http://schemas.xmlsoap.org/soap/http"
/>
24.
<operation name=
"HelloWorld"
>
25.
<soap:operation soapAction=
"http://localhost/nusoap/WebServiceServer.php/HelloWorld"
style=
"rpc"
/>
26.
<input>
27.
<soap:body
use
=
"encoded"
namespace=
""
encodingStyle=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
28.
</input>
29.
<output>
30.
<soap:body
use
=
"encoded"
namespace=
""
encodingStyle=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
31.
</output>
32.
</operation>
33.
</binding>
34.
<service name=
"HelloWorld"
>
35.
<port name=
"HelloWorldPort"
binding=
"tns:HelloWorldBinding"
>
36.
<soap:address location=
"http://localhost/nusoap/WebServiceServer.php"
/>
37.
</port>
38.
</service>
39.
</definitions>
Web Service ฝั่ง Client
สร้างไฟล์สำหรับ Client เพื่อทดสอบเรียก Web Sevice
WebServiceClient.php
01.
<html>
02.
<head>
03.
<title>ThaiCreate.Com</title>
04.
</head>
05.
<body>
06.
<?
07.
require_once
(
"lib/nusoap.php"
);
08.
$client
=
new
nusoap_client(
"http://localhost/nusoap/WebServiceServer.php?wsdl"
,true);
09.
$params
=
array
(
10.
'strName'
=>
"Weerachai Nukitram"
,
11.
'strEmail'
=>
"is_php@hotmail.com"
12.
);
13.
$data
=
$client
->call(
"HelloWorld"
,
$params
);
14.
echo
$data
;
15.
?>
16.
</body>
17.
</html>
ในตัวอย่าง มีการระบุ URL ของ Web Service ในส่วนของ new nusoap_client
// HelloWorld คือ Method ที่อยู่ใน Web Service
// $params คือ argument หรือ parameter ที่จะต้องส่งไป
เมื่อลอง echo $data; ก็จะได้สิ่งที่ Web Service ตอบกลับมา ซึ่งอาจจะอยู่ในรูปแบบของ XML หรือ String
Screenshot
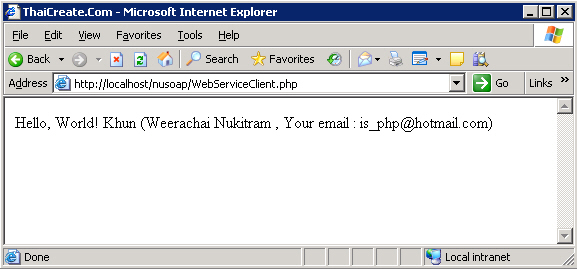
Download Code !!
ตัวอย่างที่ 2 ใช้ Soap
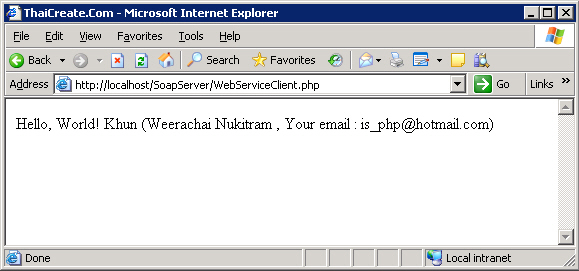
วิธีนี้จะใช้ Class ที่ชื่อว่า SoapServer ที่อยู่ใน php_soap.dll วิธีใช้งานจะต้องดาวน์โหลด extension ตัวนี้มาติดตั้งก่อน
Download php_soap.dll
หลังจากดาวน์โหลดแล้วให้แตกไฟล์และ Copy ไปไว้ในโฟเดอร์ของ extension ถ้าอยากรู้ว่าอยู่ที่ไหน ก็ให้เปิดไฟล์ php.ini
C:\Windows\php.ini
extension_dir = "D:/AppServ\php5\ext"
หาบรรทัดที่มีคำว่า extension_dir ซึ่งจะบอกว่า Path ของ extension ถูกจัดเก็บไว้ที่ไหน
หลังจาก Copy เรียบร้อยแล้วให้เพิ่มบรรทัดนี้ใน php.ini
C:\Windows\php.ini
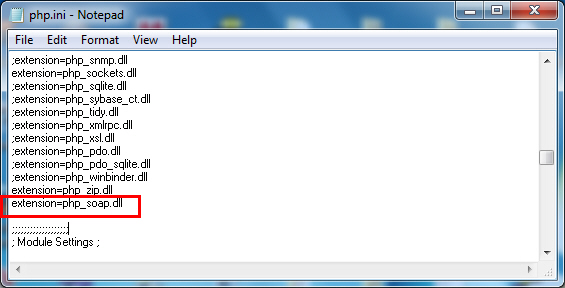
หรือดูภาพประกอบ
หลังจากแก้ไขเรียบร้อยแล้วให้ Restart Web Server หรือ Apache ซะ 1 ครั้ง
กรณี Error Class ของ SoapClient
เริ่มต้นการสร้าง Web Service ด้วย Soap
ขั้นตอนแรกให้สร้างไฟล์ helloworld.wsdl
helloworld.wsdl
01.
<?xml version=
"1.0"
encoding=
"ISO-8859-1"
?>
02.
<definitions xmlns:SOAP-ENV=
"http://schemas.xmlsoap.org/soap/envelope/"
03.
xmlns:xsd=
"http://www.w3.org/2001/XMLSchema"
04.
xmlns:xsi=
"http://www.w3.org/2001/XMLSchema-instance"
05.
xmlns:SOAP-ENC=
"http://schemas.xmlsoap.org/soap/encoding/"
06.
xmlns:tns=
"http://localhost/SoapServer/HelloWorld"
07.
xmlns:soap=
"http://schemas.xmlsoap.org/wsdl/soap/"
08.
xmlns:wsdl=
"http://schemas.xmlsoap.org/wsdl/"
09.
xmlns=
"http://schemas.xmlsoap.org/wsdl/"
10.
targetNamespace=
"http://localhost/soap/HelloWorld"
>
11.
<types>
12.
<xsd:schema targetNamespace=
"http://localhost/soap/HelloWorld"
>
13.
<xsd:import namespace=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
14.
<xsd:import namespace=
"http://schemas.xmlsoap.org/wsdl/"
/>
15.
</xsd:schema>
16.
</types>
17.
18.
<message name=
"HelloWorldRequest"
>
19.
<part name=
"strName"
type=
"xsd:string"
/>
20.
<part name=
"strEmail"
type=
"xsd:string"
/>
21.
</message>
22.
23.
<message name=
"HelloWorldResponse"
>
24.
<part name=
"return"
type=
"xsd:string"
/>
25.
</message>
26.
27.
<portType name=
"HelloWorldPortType"
>
28.
<operation name=
"HelloWorld"
>
29.
<input message=
"tns:HelloWorldRequest"
/>
30.
<output message=
"tns:HelloWorldResponse"
/>
31.
</operation>
32.
</portType>
33.
34.
<binding name=
"HelloWorldBinding"
type=
"tns:HelloWorldPortType"
>
35.
<soap:binding style=
"rpc"
transport=
"http://schemas.xmlsoap.org/soap/http"
/>
36.
<operation name=
"HelloWorld"
>
37.
<soap:operation soapAction=
"http://localhost/SoapServer/WebServiceServer.php/HelloWorld"
style=
"rpc"
/>
38.
<input>
39.
<soap:body
use
=
"encoded"
namespace=
""
encodingStyle=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
40.
</input>
41.
<output>
42.
<soap:body
use
=
"encoded"
namespace=
""
encodingStyle=
"http://schemas.xmlsoap.org/soap/encoding/"
/>
43.
</output>
44.
</operation>
45.
</binding>
46.
47.
<service name=
"HelloWorld"
>
48.
<port name=
"HelloWorldPort"
binding=
"tns:HelloWorldBinding"
>
49.
<soap:address location=
"http://localhost/SoapServer/WebServiceServer.php"
/>
50.
</port>
51.
</service>
52.
</definitions>
สำหรับไฟล์ wsdl อ่านรายละเอียดได้ที่
http://oreilly.com/catalog/webservess/chapter/ch06.html
http://www.basic-skill.com/content.php?cont_id=74&sec_id=3
สร้างไฟล์ Web Service ฝั่ง Server
WebServiceServer.php
01.
<?php
02.
$server
=
new
SoapServer(
"helloworld.wsdl"
);
03.
04.
function
HelloWorld(
$strName
,
$strEmail
){
05.
return
"Hello, World! Khun ($strName , Your email : $strEmail) "
;
06.
}
07.
08.
$server
->AddFunction(
"HelloWorld"
);
09.
$server
->handle();
10.
?>
Save ไฟล์ไว้ที่ \SoapServer\WebServiceServer.php อยู่ภายใต้ root ของ Server
ทดสอบรัน URL ของ Web Service
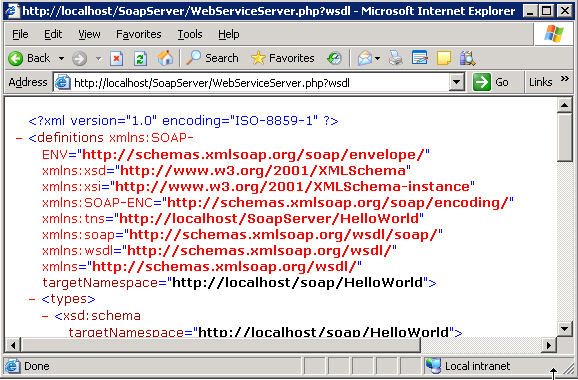
ผลลพัทธ์ที่ได้เมื่อทอสอบรัน URL ของ Web Service
Web Service ฝั่ง Client
WebServiceClient.php
01.
<html>
02.
<head>
03.
<title>ThaiCreate.Com</title>
04.
</head>
05.
<body>
06.
<?
07.
$client
=
new
SoapClient(
"http://localhost/SoapServer/WebServiceServer.php?wsdl"
,
08.
array
(
09.
"trace"
=> 1,
// enable trace to view what is happening
10.
"exceptions"
=> 0,
// disable exceptions
11.
"cache_wsdl"
=> 0)
// disable any caching on the wsdl, encase you alter the wsdl server
12.
);
13.
14.
$params
=
array
(
15.
'strName'
=>
"Weerachai Nukitram"
,
16.
'strEmail'
=>
"is_php@hotmail.com"
17.
);
18.
19.
$data
=
$client
->HelloWorld(
$params
);
20.
21.
print_r(
$data
);
22.
?>
23.
</body>
24.
</html>
ทดสอบการเรียก URL ของ Web Service ฝั่ง Client
Screenshot
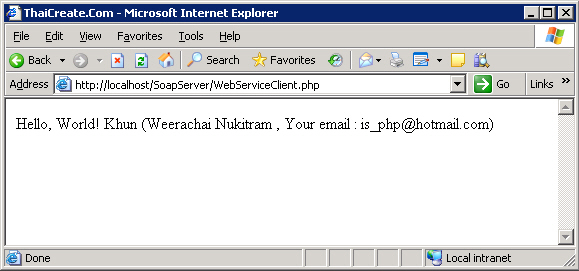
Download Code !!
บทความนี้เป็นเพียงพื้นฐานการสร้าง Web Service แบบง่าย ๆ แต่ทั้งนี้สามารถนำไปประยุกต์กับการใช้งานที่ซับซ้อน เช่นการใช้งานร่วมกับ MySQL ซึ่งไว้มีโอกาสจะลองเขียนบทความให้หลากหลายเพื่อเป็นตัวอย่างไว้ให้ศึกษา
สรุปถ้าจะให้เลือกใช้ระหว่าง NuSoap และ Soap ผมแนะนำให้ใช้ NuSoap ดีกว่า เพราะใช้งานและเข้าใจง่ายกกว่าครับ
และผิดพลาดประการใดขออภัยมา ณ ที่นี้ด้วยครับ